mirror of
https://github.com/terribleplan/next.js.git
synced 2024-01-19 02:48:18 +00:00
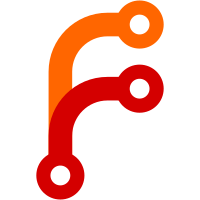
- Replaces taskr-babel with taskr-typescript for the `next` package - Makes sure Node 8+ is used, no unneeded transpilation - Compile Next.js client side files through babel the same way pages are - Compile Next.js client side files to esmodules, not commonjs, so that tree shaking works. - Move error-debug.js out of next-server as it's only used/require in development - Drop ansi-html as dependency from next-server - Make next/link esmodule (for tree-shaking) - Make next/router esmodule (for tree-shaking) - add typescript compilation to next-server - Remove last remains of Flow - Move hoist-non-react-statics to next, out of next-server - Move htmlescape to next, out of next-server - Remove runtime-corejs2 from next-server
35 lines
1,002 B
JavaScript
35 lines
1,002 B
JavaScript
/* eslint-env jest */
|
|
|
|
import shallowEquals from 'next-server/dist/lib/router/shallow-equals'
|
|
|
|
describe('Shallow Equals', () => {
|
|
it('should be true if both objects are the same', () => {
|
|
const a = { aa: 10 }
|
|
expect(shallowEquals(a, a)).toBe(true)
|
|
})
|
|
|
|
it('should be true if both objects are similar', () => {
|
|
const a = { aa: 10, bb: 30 }
|
|
const b = { aa: 10, bb: 30 }
|
|
expect(shallowEquals(a, b)).toBe(true)
|
|
})
|
|
|
|
it('should be false if objects have different keys', () => {
|
|
const a = { aa: 10, bb: 30 }
|
|
const b = { aa: 10, cc: 50 }
|
|
expect(shallowEquals(a, b)).toBe(false)
|
|
})
|
|
|
|
it('should be false if objects have same keys but different values', () => {
|
|
const a = { aa: 10, bb: 30 }
|
|
const b = { aa: 10, bb: 50 }
|
|
expect(shallowEquals(a, b)).toBe(false)
|
|
})
|
|
|
|
it('should be false if objects matched deeply', () => {
|
|
const a = { aa: 10, bb: { a: 10 } }
|
|
const b = { aa: 10, bb: { a: 10 } }
|
|
expect(shallowEquals(a, b)).toBe(false)
|
|
})
|
|
})
|