mirror of
https://github.com/terribleplan/next.js.git
synced 2024-01-19 02:48:18 +00:00
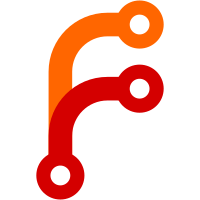
* extract request login from auth * add clarification that the monorepo is for deploy in Now only and fix typo * Refactor HOC - add authorization to HOC - add displayName to HOC - remove unnecessary `run`s in local routing
77 lines
1.7 KiB
JavaScript
77 lines
1.7 KiB
JavaScript
import Router from 'next/router'
|
|
import fetch from 'isomorphic-unfetch'
|
|
import nextCookie from 'next-cookies'
|
|
import Layout from '../components/layout'
|
|
import { withAuthSync } from '../utils/auth'
|
|
|
|
const Profile = props => {
|
|
const { name, login, bio, avatarUrl } = props.data
|
|
|
|
return (
|
|
<Layout>
|
|
<img src={avatarUrl} alt='Avatar' />
|
|
<h1>{name}</h1>
|
|
<p className='lead'>{login}</p>
|
|
<p>{bio}</p>
|
|
|
|
<style jsx>{`
|
|
img {
|
|
max-width: 200px;
|
|
border-radius: 0.5rem;
|
|
}
|
|
|
|
h1 {
|
|
margin-bottom: 0;
|
|
}
|
|
|
|
.lead {
|
|
margin-top: 0;
|
|
font-size: 1.5rem;
|
|
font-weight: 300;
|
|
color: #666;
|
|
}
|
|
|
|
p {
|
|
color: #6a737d;
|
|
}
|
|
`}</style>
|
|
</Layout>
|
|
)
|
|
}
|
|
|
|
Profile.getInitialProps = async ctx => {
|
|
const { token } = nextCookie(ctx)
|
|
const protocol = process.env.NODE_ENV === 'production' ? 'https' : 'http'
|
|
|
|
const apiUrl = process.browser
|
|
? `${protocol}://${window.location.host}/api/profile.js`
|
|
: `${protocol}://${ctx.req.headers.host}/api/profile.js`
|
|
|
|
const redirectOnError = () =>
|
|
process.browser
|
|
? Router.push('/login')
|
|
: ctx.res.writeHead(301, { Location: '/login' })
|
|
|
|
try {
|
|
const response = await fetch(apiUrl, {
|
|
credentials: 'include',
|
|
headers: {
|
|
'Content-Type': 'application/json',
|
|
Authorization: JSON.stringify({ token })
|
|
}
|
|
})
|
|
|
|
if (response.ok) {
|
|
return await response.json()
|
|
} else {
|
|
// https://github.com/developit/unfetch#caveats
|
|
return redirectOnError()
|
|
}
|
|
} catch (error) {
|
|
// Implementation or Network error
|
|
return redirectOnError()
|
|
}
|
|
}
|
|
|
|
export default withAuthSync(Profile)
|