mirror of
https://github.com/terribleplan/next.js.git
synced 2024-01-19 02:48:18 +00:00
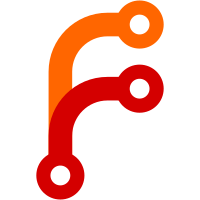
Fixes #5352 . This updates the example updating react-i18next to v8.0.6, replacing the `translate` HOC to `withNamespaces` and `I18n` to `NamespacesConsumer`. There is one thing that I am not sure if is correct or not so I need some guidance. You gotta wrap the page with the `withI18next` HOC so it will extend the `getInitialProps` of the page with this: ``` Extended.getInitialProps = async (ctx) => { const composedInitialProps = ComposedComponent.getInitialProps ? await ComposedComponent.getInitialProps(ctx) : {} const i18nInitialProps = ctx.req ? i18n.getInitialProps(ctx.req, namespaces) : {} return { ...composedInitialProps, ...i18nInitialProps } } ``` The problem lies in `i18n.getInitialProps` that has this code: ``` i18n.getInitialProps = (req, namespaces) => { if (!namespaces) namespaces = i18n.options.defaultNS if (typeof namespaces === 'string') namespaces = [namespaces] req.i18n.toJSON = () => null // do not serialize i18next instance and send to client const initialI18nStore = {} req.i18n.languages.forEach((l) => { initialI18nStore[l] = {} namespaces.forEach((ns) => { initialI18nStore[l][ns] = (req.i18n.services.resourceStore.data[l] || {})[ns] || {} }) }) return { i18n: req.i18n, // use the instance on req - fixed language on request (avoid issues in race conditions with lngs of different users) initialI18nStore, initialLanguage: req.i18n.language } } ``` In my understanding, among other things, it gets the `i18n` object from the request (included by the `server.js`) and uses the data to create `initialI18nStore` and `initialLanguage`, and then return these two objects plus the `i18n` object itself. If you add the `i18n` object on the return, then there will be a crash on the client-side render of the page: ```TypeError: Cannot read property 'ready' of null``` I don't know why, but returning it breaks `NamespacesConsumer` component from `react-i18next` (the state becomes null). So I commented this line and the provider on `_app.js` is getting the `i18n` instance from the `i18n.js` file (the same as `server.js`). I don't know if this would be an issue so I would like help to debug this.
60 lines
1.7 KiB
JavaScript
60 lines
1.7 KiB
JavaScript
const i18n = require('i18next')
|
|
const XHR = require('i18next-xhr-backend')
|
|
const LanguageDetector = require('i18next-browser-languagedetector')
|
|
|
|
const options = {
|
|
fallbackLng: 'en',
|
|
load: 'languageOnly', // we only provide en, de -> no region specific locals like en-US, de-DE
|
|
|
|
// have a common namespace used around the full app
|
|
ns: ['common'],
|
|
defaultNS: 'common',
|
|
|
|
debug: false, // process.env.NODE_ENV !== 'production',
|
|
saveMissing: true,
|
|
|
|
interpolation: {
|
|
escapeValue: false, // not needed for react!!
|
|
formatSeparator: ',',
|
|
format: (value, format, lng) => {
|
|
if (format === 'uppercase') return value.toUpperCase()
|
|
return value
|
|
}
|
|
}
|
|
}
|
|
|
|
// for browser use xhr backend to load translations and browser lng detector
|
|
if (process.browser) {
|
|
i18n
|
|
.use(XHR)
|
|
// .use(Cache)
|
|
.use(LanguageDetector)
|
|
}
|
|
|
|
// initialize if not already initialized
|
|
if (!i18n.isInitialized) i18n.init(options)
|
|
|
|
// a simple helper to getInitialProps passed on loaded i18n data
|
|
i18n.getInitialProps = (req, namespaces) => {
|
|
if (!namespaces) namespaces = i18n.options.defaultNS
|
|
if (typeof namespaces === 'string') namespaces = [namespaces]
|
|
|
|
req.i18n.toJSON = () => {} // do not serialize i18next instance to prevent circular references on the client
|
|
|
|
const initialI18nStore = {}
|
|
req.i18n.languages.forEach((l) => {
|
|
initialI18nStore[l] = {}
|
|
namespaces.forEach((ns) => {
|
|
initialI18nStore[l][ns] = (req.i18n.services.resourceStore.data[l] || {})[ns] || {}
|
|
})
|
|
})
|
|
|
|
return {
|
|
i18n: req.i18n, // use the instance on req - fixed language on request (avoid issues in race conditions with lngs of different users)
|
|
initialI18nStore,
|
|
initialLanguage: req.i18n.language
|
|
}
|
|
}
|
|
|
|
module.exports = i18n
|